How Does Python Development Work?
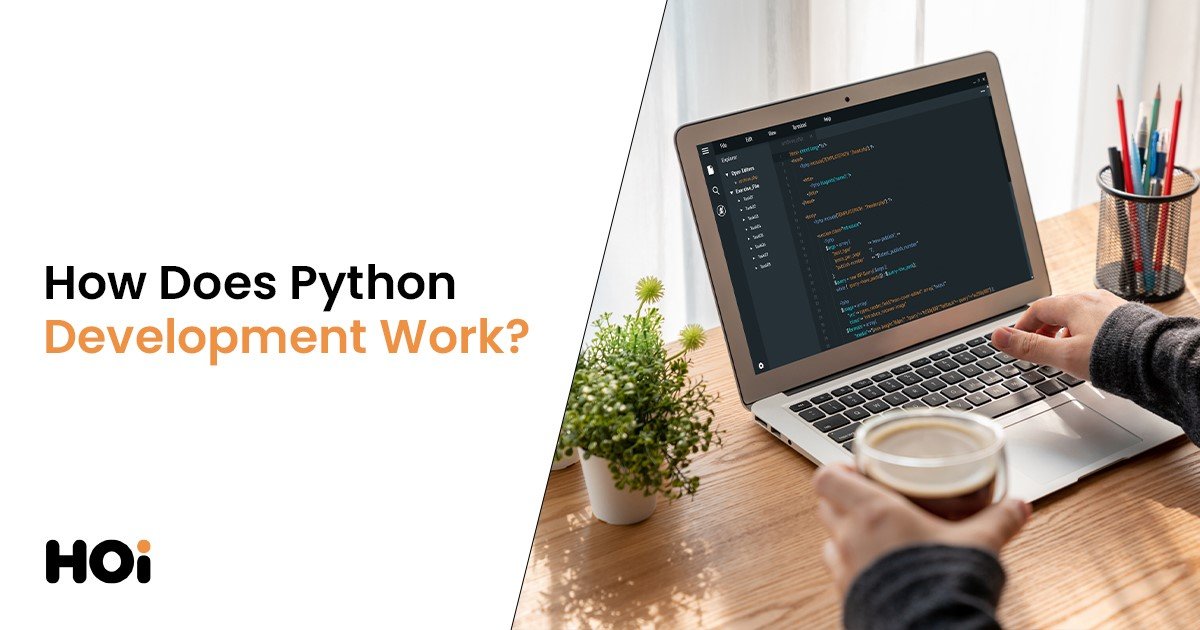
Introduction
Python is a widely used excessive-degree programming language famous among developers for its simplicity, readability, and flexibility. Created by Guido van Rossum and first released in 1991, Python’s straightforward syntax permits novices to hold close programming principles quickly while presenting pro developers with practical tools to construct complicated programs. From internet improvement to facts science and system learning, Python’s widespread surroundings of libraries and frameworks support a considerable array of use cases.
Purpose of the Blog
This weblog is designed to delve into the core factors of Python improvement, supplying realistic insights and pointers. We’ll cover topics that include putting in place improvement surroundings, the fundamentals of Python programming, famous libraries and frameworks, acceptable practices, and not-unusual workflows. Whether you’re a Python amateur or seeking to enhance your capabilities, this guide will equip you with the expertise you need to excel in your Python adventure.
What is Python?
History of Python
Python is an excessive-level programming language created by Guido van Rossum and first launched in 1991. Its origins may be traced back to the late Eighties, when van Rossum, stimulated by the ABC programming language, started developing Python as a successor that could improve on its predecessor’s limitations.
Characteristics of Python
Python quickly received recognition for its focus on simplicity and clarity, using sizable whitespace and a clean syntax that makes it approachable for beginners and skilled developers alike. This emphasis on readability, mixed with Python’s versatility throughout numerous domain names—from net improvement and automation to records science and synthetic intelligence—has contributed to its tremendous adoption.
Python is not just a language; it’s a vibrant community. This community contributes to a rich ecosystem of libraries, frameworks, and tools, making Python a top choice for many developers.
Setting Up a Python Development Environment
Installing Python
Setting up a Python development environment is the first step in your journey into the world of Python programming. To start, you’ll need to install Python on your system, and the process varies slightly depending on your operating system. On Windows, you can download the installer from the official Python website and run it, ensuring you check the option to add Python to your system’s PATH.
Python is typically pre-installed for macOS, but updating to the latest version from the website or through Homebrew is often recommended. Linux users can install Python using their system’s package manager (like `apt` for Debian-based systems). After installation, it’s essential to confirm that Python and `pip` (Python’s package manager) are correctly installed by opening a command line or terminal and running `python –version` and `pip –version. `
Integrated Development Environments (IDEs)
Once Python is set up, you can choose an Integrated Development Environment (IDE) to make coding more accessible and efficient. Popular IDEs for Python development include PyCharm, which is feature-rich and suitable for professional developers; Visual Studio Code, known for its versatility and extensions; and Jupyter Notebooks, ideal for data science and interactive computing. After selecting your IDE, you can start creating Python projects and writing code.
Package Management with `pip`
Finally, package management with `pip` allows you to install and manage external Python libraries and modules. To install a package, you use the command `pip install <package_name>. ` For example, you would run `pip install pandas` to install the popular data analysis library Pandas.
This flexibility in installing packages makes Python a robust and adaptable language that supports various applications, from web development to data science. The strong community support ensures that Python remains relevant and continues to evolve, making it a reliable choice for your programming needs.
Core Concepts in Python Programming
Basic Syntax and Structure
Python’s syntax is designed for readability, using indentation to define code blocks instead of braces or keywords. This emphasis on consistent indentation fosters clear and maintainable code. Comments are crucial for explaining code logic, and Python supports both single-line (`#`) and multi-line comments (`”…”`). Naming conventions in Python typically follow snake_case for variable and function names and CamelCase for class names.
Data Types and Structures
Python offers diverse data types, allowing developers to choose the most appropriate structures for their needs. Basic types include integers, floats, strings, and Booleans. Python’s complex data structures, such as lists, tuples, sets, and dictionaries, provide flexibility for organizing and manipulating data. Lists are ordered and mutable, while tuples are immutable and suitable for fixed collections. Dictionaries with key-value pairs are perfect for associative data storage and retrieval.
Control Flow Statements
Control flow in Python is managed through loops, conditionals, and exception handling. The `for` loop iterates over iterable objects like lists or ranges, while the `while` loop continues if a specified condition holds. Conditional statements, including `if,` `else,` and `elif,` enable branching logic to handle different scenarios. Python’s `try-except` structure allows for graceful error handling, letting developers catch and manage exceptions to prevent program crashes. These control flow tools are essential for creating flexible and robust Python applications.
Writing Python Code
Functions and Modules
Creating reusable and modular code is a core aspect of Python development. Functions in Python allow you to define blocks of code that can be called by name and reused throughout your program. To create a function, use the `def` keyword, followed by a function name and any parameters it accepts. After defining the function, you can call it from other parts of your code to perform specific tasks.
Modules extend this concept by grouping related functions, classes, and variables into separate files. Using the ‘ import ‘ statement, you can import these modules into your code, allowing you to reuse code across multiple scripts. This modular approach enhances code organization and maintainability.
Object-Oriented Programming (OOP)
Python supports Object-Oriented Programming (OOP), enabling developers to create classes that serve as blueprints for objects. A class can contain attributes (variables) and methods (functions), providing a structured model for real-world entities and their behaviors.
To define a class, use the `class` keyword, then create instances (objects) from the class. Python’s OOP features include concepts like inheritance, encapsulation, and polymorphism, allowing for complex and extensible applications. This paradigm is instrumental in large-scale projects where modularity and reusability are essential.
File Handling
File handling in Python is simple and versatile, allowing you to read from and write to files with various methods. The built-in `open` function lets you open files in different modes, such as ‘r’ for reading, ‘w’ for writing, and ‘a’ for appending. You can read a file’s content line by line or as a whole and write new content or overwrite existing data as needed. Properly closing the file with `close()` or using a `with` statement ensures that resources are appropriately managed and prevents file-related issues. This flexibility in file handling makes Python suitable for a wide range of applications, from data processing to configuration management and beyond.
Python Libraries and Frameworks
Popular Libraries
Python’s wealthy environment of libraries is an excellent purpose for its massive reputation in various fields. Among the most distinguished libraries, NumPy stands out for its strong support for massive-scale numerical computations and array operations. With intuitive information systems like DataFrames, Pandas is a move-to device for fact manipulation and evaluation. Regarding visualizing facts, Matplotlib gives a versatile plotting library, often used along with other libraries like Seaborn for extra complex visualizations.
Web Frameworks
In web improvement, Python offers robust frameworks like Django and Flask. Django provides a high-stage framework for constructing scalable net applications with built-in admin panels, authentication structures, and a sturdy ORM. On the other hand, Flask offers a lightweight and flexible approach, ideal for smaller initiatives or those requiring greater customization.
Data Science and Machine Learning
Due to its substantial libraries, Python has won tremendous traction in information technology and device mastering. TensorFlow and PyTorch are the main frameworks that permit builders to construct sophisticated fashions for obligations, from easy categories to superior neural networks. These libraries are frequently used along facts-centered gear like Pandas and Matplotlib, growing a robust environment for facts-pushed projects.
Best Practices in Python Development
Code Readability and PEP 8
Code readability is a cornerstone of Python improvement and the PEP 8 suggestions function as a comprehensive manual to ensure regular and smooth code. PEP 8, or Python Enhancement Proposal 8, outlines pleasant practices for code style, including proper indentation, naming conventions, and import ordering. Following these guidelines makes your code more maintainable and fosters collaboration among builders.
Testing and Debugging
Testing and debugging are similarly vital in Python development. Frameworks like `unittest` and `pytest` offer robust equipment for creating check cases and automating the testing process, supporting developers in identifying and resolving problems early in the improvement cycle. Effective debugging strategies, which include using breakpoints and print statements, can also aid in quickly pinpointing and solving mistakes, ensuring a smoother improvement workflow.
Version Control with Git
Version control is essential for handling code adjustments, monitoring revisions, and facilitating collaborative work on tasks. Git is the leading version of the manipulated device, presenting developers with the capability to department, merge, and preserve a clear history of code evolution.
This flexibility makes it less complicated to paint in groups, experiment with new features without danger, and revert adjustments when necessary. By adopting those excellent practices, Python developers can create fantastic code and hold a collaborative and green development environment.
Python Development Workflow
Development Stages
The Python improvement workflow encompasses several vital stages that manualize a venture from conception to deployment. It starts with the planning stage, in which developers define the undertaking’s scope, necessities, and architecture. This is observed through the improvement level, in which code is written, modules are incorporated, and functionality is applied. The following testing stage ensures the code works as predicted and identifies any insects or issues. This stage regularly involves unit checking out, integration trying out, and person acceptance trying out. Finally, the deployment degree entails releasing the software to production surroundings, wherein customers may use it.
Continuous Integration/Continuous Deployment (CI/CD)
Many development teams adopt Continuous Integration/Continuous Deployment (CI/CD) practices to streamline those levels. CI includes automating the combination of code modifications right into a shared repository, allowing teams to hit upon troubles early and preserve a regular construct. CD extends this concept by automating the deployment procedure, decreasing the time between code modifications and deployment to production. This automation fosters an extra agile improvement and helps teams release updates and new features more frequently.
Collaborative Development
Collaboration is essential throughout the workflow, mainly in team-based tasks. Tools like Git facilitate model manipulation and collaborative coding, permitting developers to work on separate branches, merge code, and make music adjustments. Teams also use systems like GitHub or GitLab to manage projects, conduct code reviews, and discuss venture-associated troubles. By combining these practices, Python improvement teams can create a seamless and green workflow, ultimately turning in first-rate applications.
Conclusion
We’ve protected the essential components of Python development, from setting up a robust improvement environment to grasping core principles like capabilities, modules, and object-oriented programming. We’ve additionally touched on famous Python libraries, excellent practices, and effective workflows, which are essential for any Python developer. We endorse exploring Python documentation and online sources like Codecademy, Coursera, or Real Python for those seeking to deepen their understanding.
If you’re ready to take your Python skills to the next level and collaborate with experts in the field, we invite you to work with us. Our team specializes in Python-based projects and is always excited to welcome new talent and ideas. Whether you’re an experienced developer or just starting, we’re here to help you grow. Get in touch, and let’s create something unique together!
FAQs
1. How do I set up a Python development environment?
To set up a Python development environment, start by installing Python from the official website or using a package manager. Confirm the installation by checking the versions with python –version and pip –version. Choose an Integrated Development Environment (IDE) such as PyCharm, Visual Studio Code, or Jupyter Notebooks for coding. Manage external libraries with pip, for example, using pip install pandas to add packages as needed.
2. What are some key features of Python?
Python is praised for its readability and simplicity, using indentation for clear, maintainable code. Its versatility spans various fields like web development, data science, and machine learning. The language benefits from a strong community and a rich ecosystem of libraries and frameworks, including NumPy for numerical tasks, Django for web development, and TensorFlow for machine learning.
3. What are best practices for Python development?
Best practices in Python development include following PEP 8 guidelines for code readability, using testing frameworks like unit test or pytest to catch issues early, and employing Git for version control to manage code changes and collaboration effectively. These practices help ensure high-quality, maintainable code and a streamlined development process.